Integrating Selenium WebDriver with Pytest for Efficient Testing
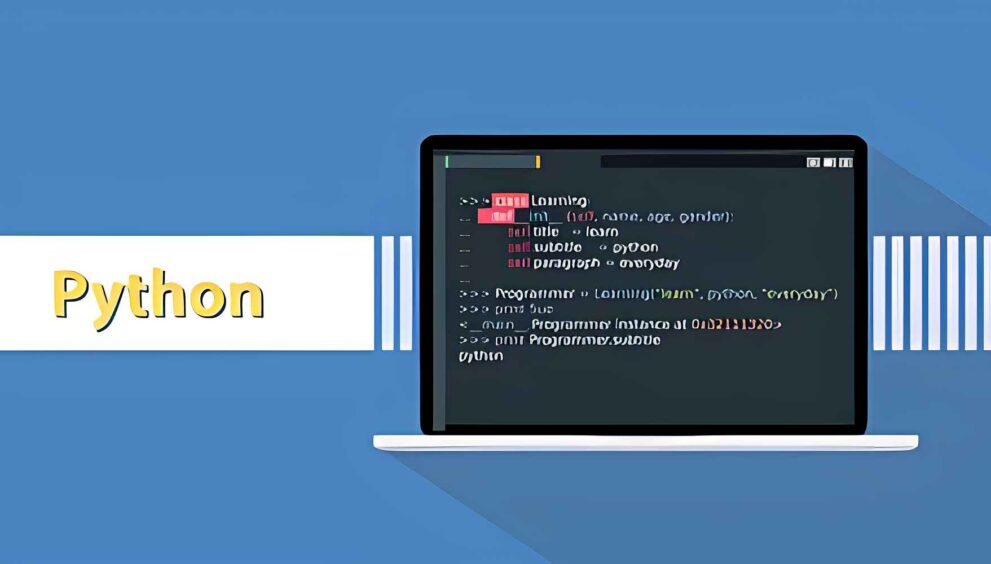
Integrating Selenium WebDriver with Pytest for Efficient Testing
In the world of software development, testing is a crucial step to ensure the quality and reliability of an application. Automation testing has gained immense popularity due to its efficiency and effectiveness in identifying bugs and issues early in the development process. One of the popular tools used for automation testing is Selenium WebDriver, and when combined with Pytest, a powerful testing framework for Python, it can greatly enhance the testing process. In this article, we will explore how to integrate Selenium WebDriver with Pytest for efficient testing.
Table of Contents
Sr# | Headings |
1. | Introduction to Selenium WebDriver and Pytest |
2. | Setting Up Selenium WebDriver and Pytest Environment |
3. | Writing Your First Test Case with Selenium WebDriver and Pytest |
4. | Organizing Test Cases with Pytest |
5. | Running Tests with Pytest |
6. | Parameterizing Tests with Pytest |
7. | Handling Test Fixtures with Pytest |
8. | Best Practices for Integration |
9. | Conclusion |
1. Introduction to Selenium WebDriver and Pytest
Selenium WebDriver is a powerful tool for automating web application testing across different browsers. It provides a simple and concise API for interacting with web elements and simulating user interactions. On the other hand, Pytest is a testing framework for Python that makes it easy to write simple and scalable test cases. When combined, Automation with Python and Pytest offer a robust solution for automated testing.
2. Setting Up Selenium WebDriver and Pytest Environment
To start using Selenium WebDriver with Pytest in automation python , you need to install the necessary packages. You can install Selenium WebDriver using pip:
bash
Copy code
pip install selenium
For Pytest, you can install it using:
bash
Copy code
pip install pytest
You also need to download the appropriate WebDriver for the browser you intend to test (e.g., ChromeDriver for Google Chrome).
3. Writing Your First Test Case with Selenium WebDriver and Pytest
Here’s a simple example of a test case using Selenium WebDriver and Pytest to open a website and assert its title:
python
Copy code
import pytest
from selenium import webdriver
@pytest.fixture(scope=”module”)
def driver():
driver = webdriver.Chrome()
yield driver
driver.quit()
def test_website_title(driver):
driver.get(“https://www.example.com”)
assert driver.title == “Example Domain”
4. Organizing Test Cases with Pytest
Pytest allows you to organize your test cases into modules and classes for better management. You can use fixtures to set up and tear down resources needed for testing.
5. Running Tests with Pytest
You can run your tests using the following command:
bash
Copy code
pytest test_file.py
Pytest will automatically discover and run your test cases, displaying the results in a user-friendly format.
6. Parameterizing Tests with Pytest
Pytest allows you to parameterize your test cases, enabling you to run the same test with different inputs. This can be useful for testing different scenarios.
7. Handling Test Fixtures with Pytest
Pytest provides powerful fixtures for managing test setup and teardown. You can use fixtures to create reusable setup and teardown logic for your tests.
8. Best Practices for Integration
- Keep your test cases small and focused on a single functionality.
- Use descriptive names for your test cases and fixtures.
- Use fixtures to set up and tear down resources needed for testing.
- Use parameterization to run the same test with different inputs.
- Use assertions to verify the expected outcomes of your test cases.
9. Conclusion
Integrating python for automation testing Selenium WebDriver with Pytest can greatly improve the efficiency and effectiveness of your automated testing process. By following the best practices and guidelines mentioned in this article, you can create robust and reliable test cases that help ensure the quality and reliability of your applications.
FAQs
Can I use Selenium WebDriver with Pytest for testing mobile applications?
- Yes, Selenium WebDriver can be used with Pytest for testing mobile applications using tools like Appium.
Is it possible to run Selenium WebDriver tests in parallel with Pytest?
- Yes, Pytest allows you to run tests in parallel using the -n option to specify the number of parallel processes.
How can I handle dynamic elements in Selenium WebDriver tests with Pytest?
- You can use various techniques such as waiting for the element to be present, using explicit waits, or using dynamic locators to handle dynamic elements in Selenium WebDriver tests with Pytest.
Can I integrate Selenium WebDriver with other testing frameworks besides Pytest?
- Yes, Selenium WebDriver can be integrated with other testing frameworks like unittest, Robot Framework, etc., but Pytest is popular due to its simplicity and scalability.
Is Selenium WebDriver compatible with all browsers?
- Selenium WebDriver is compatible with most major browsers, including Chrome, Firefox, Safari, and Internet Explorer, by using the appropriate WebDriver for each browser.